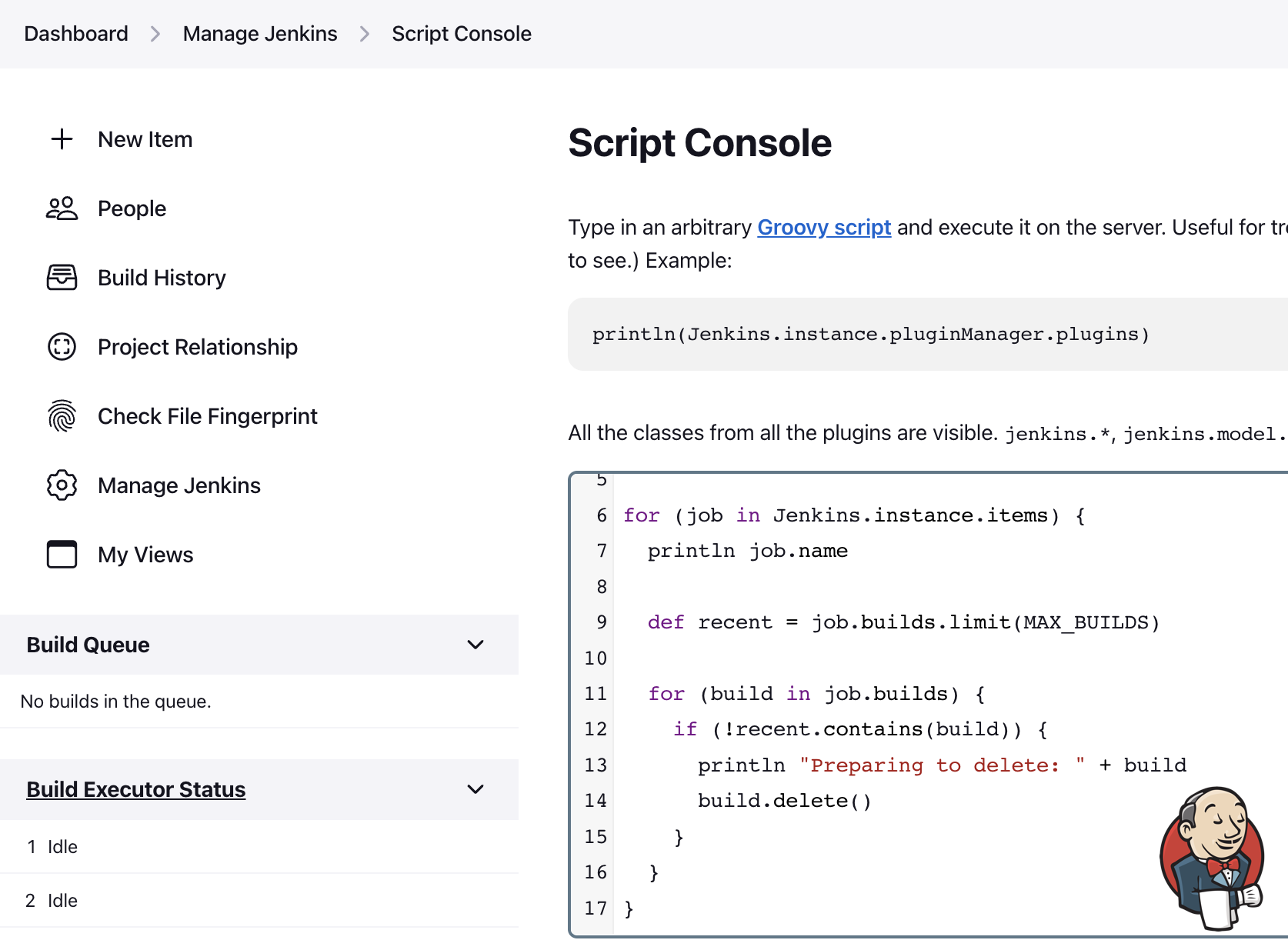
Jenkins Remove All Builds
Go to Dashboard > Manage Jenkins > Script Console and use the following script to leave just last build import jenkins.model.Jenkins import hudson.model.Job MAX_BUILDS = 1 for (job in Jenkins.instance.items) { println job.name def recent = job.builds.limit(MAX_BUILDS) for (build in job.builds) { if (!recent.contains(build)) { println "Preparing to delete: " + build build.delete() } } }